Destinations
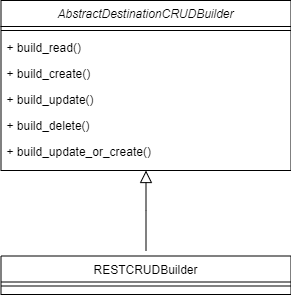
AbstractDestinationCRUDBuilder
RESTCRUDBuilder
- class crudcreator.destination.rest.RESTCRUDBuilder.RESTCRUDBuilder(
- index_filter_override: dict[str, Tuple[Any, Callable]],
Allows you to build a REST interface (FastAPI router) for several types of entities.
- Parameters:
index_filter_override (dict[str, Tuple[Any, Callable]]) –
- build_bulk_update(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
- ids_from_dependencies: dict[str, Depends] = {},
Adds a PATCH route to the router to modify multiple entities.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The type of entity on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
ids_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with an id (which will not be provided by the API user). For example, a dependency that reads a jwt token and returns the username of the user calling the route (for example, to restrict update to entities over which the user has rights).
TODO: make a generic build_bulk that builds what’s needed for update, create and delete.
- build_create(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
Adds a POST route to the router to create an entity.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The type of entity on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
- build_delete(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
- ids_from_dependencies: dict[str, Depends] = {},
Adds a DELETE route to the router to delete an entity.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The type of entity on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
ids_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with an id (which will not be provided by the API user). For example, a dependency that reads a jwt token and returns the username of the user calling the route (for example, to restrict delete to entities over which the user has rights).
- build_read(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
- filter_from_dependencies: dict[str, Depends] = {},
Adds a GET route to the router to read a list of entities.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The type of entity on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
filter_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with a filter (which will not be provided directly by the API user).
- build_update(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
- ids_from_dependencies: dict[str, Depends] = {},
Adds a PATCH route to the router to modify an entity.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The type of entity on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
ids_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with an id (which will not be provided by the API user). For example, a dependency that reads a jwt token and returns the username of the user calling the route (for example, to restrict update to entities over which the user has rights).
- build_update_or_create(
- entity_type: AbstractCRUDableEntityType,
- transaction_manager: AbstractTransactionManager,
- ids_from_dependencies: dict[str, Depends] = {},
- values_from_dependencies: dict[str, Depends] = {},
Adds a PUT route to the router to add an entity if it doesn’t exist, or modify it if it does.
- Parameters:
entity_type (AbstractCRUDableEntityType) – The entity type on which to plug the destination interface.
transaction_manager (AbstractTransactionManager) – The manager that will handle all transactional matters.
ids_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with an id (which will not be provided by the API user). For example, a dependency that reads a jwt token and returns the username of the user calling the route (for example, to restrict update to entities over which the user has rights).
values_from_dependencies (dict[str, Depends]) – A dictionary associating a dependency with a value (which will then not be provided by the API user). For example, a dependency that reads a jwt token and returns the username of the user calling the route (for example, to add the name of the user who is using the API to the entity it creates).