Entity type
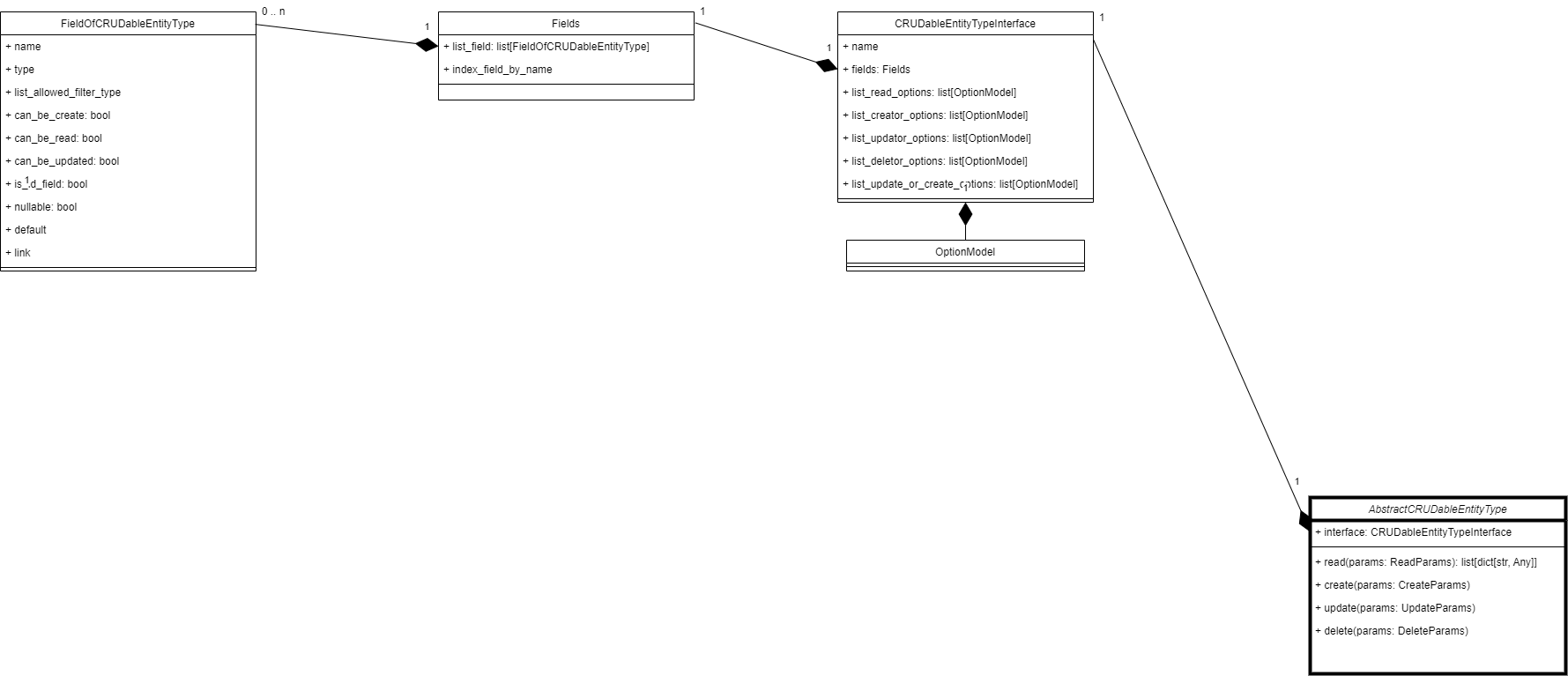
AbstractCRUDableEntityType
- pydantic model crudcreator.AbstractCRUDableEntityType.AbstractCRUDableEntityType[source]
An instance of this class represents a CRUDable entity type. This is CRUDCreator’s main class. Proxies and source modules inherit from this class. See How does it work?.
- field interface: CRUDableEntityTypeInterface [Required][source]
The interface/schema of the entity type, as seen by the entity’s CRUD users.
- async check_integrity(
- transaction: AbstractTransaction,
- dict_value_new_entity: dict[str, Any],
Used to check that a hypothetical entity, represented by dict_value_new_entity, would not violate any integrity constraints if created.
- Parameters:
transaction (AbstractTransaction) – The current transaction
dict_value_new_entity (dict[str, Any]) –
A field name -> value dictionary. Represents the fields of the new entity which we want to check that it will not violate integrity constraints if created.
Warning
Must therefore include at least all ids fields that are not automatically generated.
Warning
All mandatory filter fields must also be present in dict_value_new_entity. Otherwise, there’s a risk that a proxy read will crash, because it was expecting a filter that isn’t there.
- Raises:
EntityAlreadyExist – If the creation of an entity with “dict_value_new_entity” values would violate the ids uniqueness constraint.
- async create(
- params: CreateParams,
Creates an entity.
- Parameters:
params (CreateParams) – CRUD query parameters create.
- async delete(
- params: DeleteParams,
Deletes an entity.
- Parameters:
params (DeleteParams) – CRUD delete query parameters.
- async read(
- params: ReadParams,
Returns a list of entities. Entities are returned as dictionaries, whose keys are the entity’s attributes.
- Parameters:
params (ReadParams) – CRUD read query parameters.
- Return type:
list[dict[str, Any]]
- async update(
- params: UpdateParams,
Updates an entity.
- Parameters:
params (UpdateParams) – CRUD query parameters update.
- async update_or_create(
- params: UpdateOrCreateParams,
Creates an entity if it doesn’t exist, updates it if it does.
- Parameters:
params (UpdateOrCreateParams) – CRUD query parameters update_or_create.
CRUDableEntityTypeInterface
- pydantic model crudcreator.interface.CRUDableEntityTypeInterface.CRUDableEntityTypeInterface[source]
Represents the schema of an entity type, i.e. what is visible to CRUD users on the entity. Performs no CRUD action as such. It’s an empty shell.
- field list_creator_options: list[OptionModel] = [][source]
Customizable options for crud actions (index name->type)
- field list_deletor_options: list[OptionModel] = [][source]
Customizable options for crud actions (index name->type)
- field list_read_options: list[OptionModel] = [][source]
Customizable options for crud actions (index name->type)
- field list_update_or_create_options: list[OptionModel] = [][source]
Customizable options for crud actions (index name->type)
- field list_updator_options: list[OptionModel] = [][source]
Customizable options for crud actions (index name->type)
- get_create_options_data_model(
- prefix: str = '',
Returns the Pydantic model of options for an entity creation.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_creator_data_model(
- prefix: str = '',
Returns the Pydantic model of expected data for an entity creation.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_delete_options_data_model(
- prefix: str = '',
Returns the Pydantic model of options for an entity suppression.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_fields_can_be_created() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields the user is allowed to write.
- Return type:
- get_fields_can_be_updated() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields that the user has the right to modify.
- Return type:
- get_fields_can_be_updated_or_created() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields that the user has the right to modify or create.
- Return type:
- get_filter_data_model(
- prefix: str = '',
Returns the Pydantic model for filters.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_filterable_field() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields on which the user has the right to filter.
- Return type:
- get_ids_data_model(
- prefix: str = '',
Returns the Pydantic model of the data expected to identify a single entity.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_ids_field() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields used to uniquely identify an entity (entity identifiers).
- Return type:
- get_read_options_data_model(
- prefix: str = '',
Returns the Pydantic model of entity reading options.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_read_response_data_model(
- prefix: str = '',
Returns the Pydantic model of the entities returned by a read.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_readable_field() list[FieldOfCRUDableEntityType] [source]
Returns the list of fields the user is allowed to read.
- Return type:
- get_readable_field_name() list[str] [source]
Returns a list of field names that the user is authorized to read.
- Return type:
list[str]
- get_sub_interface(
- name: str,
- list_field_name_to_keep: list[str],
Creates and returns an interface identical to self, but contains only the list of fields to be retained. Gives a new name to the interface thus created.
- Parameters:
name (str) – The name of the new interface.
list_field_name_to_keep (list[str]) – The list of fields to keep.
- Return type:
- get_update_options_data_model(
- prefix: str = '',
Returns the Pydantic model of options for an entity update.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_update_or_create_data_model(
- prefix: str = '',
Returns the Pydantic model of the data expected for an entity “creation or update”.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
- get_update_or_create_options_data_model(
- prefix: str = '',
Returns the Pydantic model of options for an entity “creation or modification”.
- Parameters:
prefix (str) – A prefix to be added to the Pydantic model name to make it unique (necessary for swagger REST APIs, for example)
- Return type:
BaseModel
Fields
- pydantic model crudcreator.Fields.Fields[source]
An instance of this class represents the fields of an entity.
- field index_field_by_name: dict[str, FieldOfCRUDableEntityType] [Required][source]
A name -> field index built by the “build” classmethod.
- field list_field: list[FieldOfCRUDableEntityType] [Required][source]
The list of fields.
- classmethod build(
- list_field: list[FieldOfCRUDableEntityType],
Use this method to build an instance of Fields.
- Parameters:
list_field (list[FieldOfCRUDableEntityType]) – The list of fields.
FieldOfCRUDableEntityType
- pydantic model crudcreator.FieldOfCRUDableEntityType.FieldOfCRUDableEntityType[source]
An instance of this class represents a field of a CRUDable entity type.
- field can_be_created: bool | None = False[source]
Can a value for this field be specified when the entity is created? TODO : careful, update_or_create for fields with can_be_create!=can_be_updated
- field can_be_updated: bool | None = False[source]
Can a value for this field be specified when modifying the entity? TODO : careful, update_or_create for fields with can_be_create!=can_be_updated
- field default: Any | None = 'unknown'[source]
The default value of the field. If the default value is not provided, it will be filled in automatically when the program is launched, during source analysis.
- field is_automatically_generated: bool | None = 'unknown'[source]
Is this field automatically generated by the source when the entity is created? If this information is not provided, it will be filled in automatically when the program is launched during source analysis. TODO : to review. More interesting to have “uniquely_generated”?
- field is_id_field: bool | None = 'unknown'[source]
Indicates whether the field is part of the entity’s unique identifier. If this information is not provided, it will be filled in automatically when the program is launched during source analysis.
- field link: Link | None = None[source]
If the field represents a link with another field of another entity (equivalent to a foreign key in SQL).
- field list_allowed_filter_type: list[FilterType] | None = None[source]
How can entities be filtered when a filter is specified on this field?
- field nullable: bool | None = 'unknown'[source]
Indicates whether the field can be null. If this information is not provided, it will be filled in automatically when the program is launched during source analysis.
- field type: Any | None = 'unknown'[source]
Field type. If not provided, it will be filled in automatically when the program is launched, during source analysis.