Builders
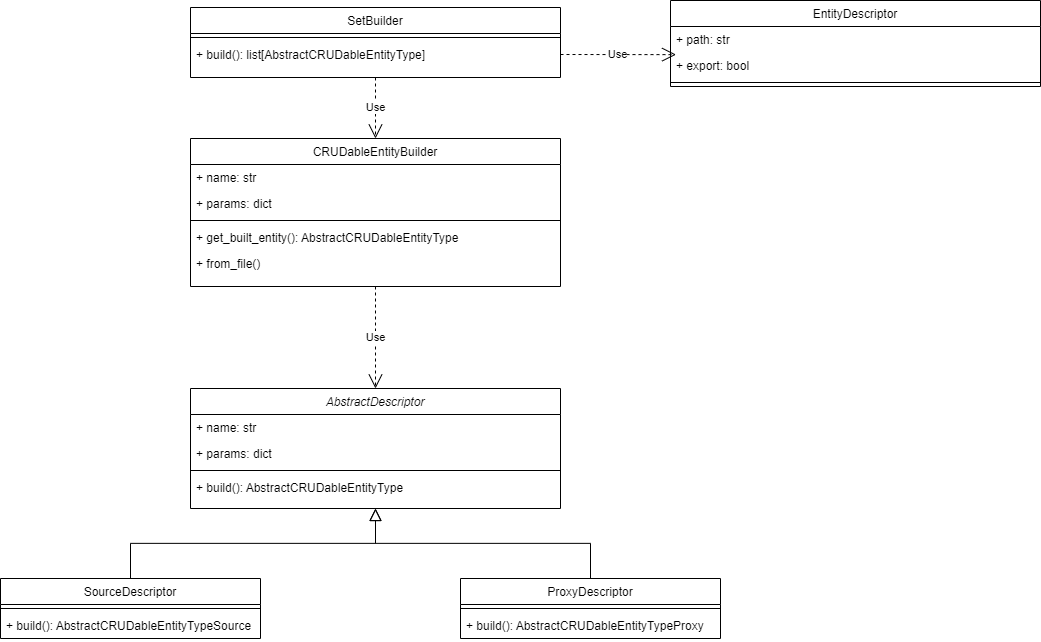
Building from .json files
CRUDableEntityBuilder
- class crudcreator.builder.CRUDableEntityBuilder.CRUDableEntityBuilder(
- crudable_source: AbstractCRUDableEntityType | None,
Enables you to build an entity type.
- Parameters:
crudable_source (AbstractCRUDableEntityType | None) –
- async from_file(
- file_path: str,
- subs_index: dict[str, Any],
- addon_source: dict[str, Type[AbstractCRUDableEntityTypeSource]],
- addon_proxy: dict[str, Type[AbstractCRUDableEntityTypeProxy]],
Builds an entity type from a .json file that lists the source and proxies.
- Parameters:
file_path (str) – The path to the .json file
subs_index (dict[str, Any]) – An index that indicates the corresponding Python object for variables in descriptors.
addon_source (dict[str, Type[AbstractCRUDableEntityTypeSource]]) – An index of the library user’s custom source modules.
addon_proxy (dict[str, Type[AbstractCRUDableEntityTypeProxy]]) – An index of the library user’s custom proxy modules.
- get_built_entity() AbstractCRUDableEntityType [source]
Returns the constructed entity.
- Return type:
SetBuilder
- class crudcreator.builder.SetBuilder.SetBuilder[source]
Allows multiple entity types to be constructed. Useful when entities refer to each other.
- async build(
- list_entity_descriptor: list[EntityDescriptor],
- subs_index: dict[str, Any],
- addon_source: dict[str, AbstractCRUDableEntityTypeSource],
- addon_proxy: dict[str, AbstractCRUDableEntityTypeProxy],
Builds multiple entity types from a list of .json files. Stores in an index references as it encounters them, which it merges with subs_index.
- Parameters:
list_entity_descriptor (list[EntityDescriptor]) – The list of json files.
subs_index (dict[str, Any]) – An index that indicates the corresponding Python object for variables in descriptors.
addon_source (dict[str, AbstractCRUDableEntityTypeSource]) – An index of the library user’s custom source modules.
addon_proxy (dict[str, AbstractCRUDableEntityTypeProxy]) – An index of the library user’s custom proxy modules.
- Return type:
Descriptors
- pydantic model crudcreator.AbstractDescriptor.AbstractDescriptor[source]
Describes a module, without having built it. Is an intermediate class used by CRUD constructors to build entity types. Library users should never have to use this class directly.
- field addons: dict[str, Type[AbstractCRUDableEntityType]] [Required][source]
Index of personal modules implemented by the crudcreator user.
- field subs_index: dict[str, Any] [Required][source]
Index of Python objects associated with variables in the descriptor.
- build() AbstractCRUDableEntityType [source]
To be overloaded. Builds the entity type associated with this descriptor.
- Return type:
- get_class() T [source]
Returns the class associated with the descriptor. Fetches the class from the index of modules pre-implemented by crudcreator. Otherwise, search in addons.
- Return type:
T
- get_index() dict[str, Any] [source]
To be overloaded. Must return the index of modules pre-implemented by the library.
- Return type:
dict[str, Any]
- pydantic model crudcreator.source.SourceDescriptor.SourceDescriptor[source]
- field addons: dict[str, Type[AbstractCRUDableEntityTypeSource]] [Required][source]
Index of personal modules implemented by the crudcreator user.
- build() AbstractCRUDableEntityTypeSource [source]
To be overloaded. Builds the entity type associated with this descriptor.
- Return type:
- get_index() AbstractCRUDableEntityTypeSource [source]
To be overloaded. Must return the index of modules pre-implemented by the library.
- Return type:
- pydantic model crudcreator.proxy.ProxyDescriptor.ProxyDescriptor[source]
- field addons: dict[str, Type[AbstractCRUDableEntityTypeProxy]] [Required][source]
Index of personal modules implemented by the crudcreator user.
- field base: AbstractCRUDableEntityType | None = None[source]
The module for which this descriptor will be the proxy.
- build() AbstractCRUDableEntityTypeProxy [source]
Builds the proxy associated with the descriptor.
- Return type:
- get_index() AbstractCRUDableEntityTypeProxy [source]
To be overloaded. Must return the index of modules pre-implemented by the library.
- Return type: